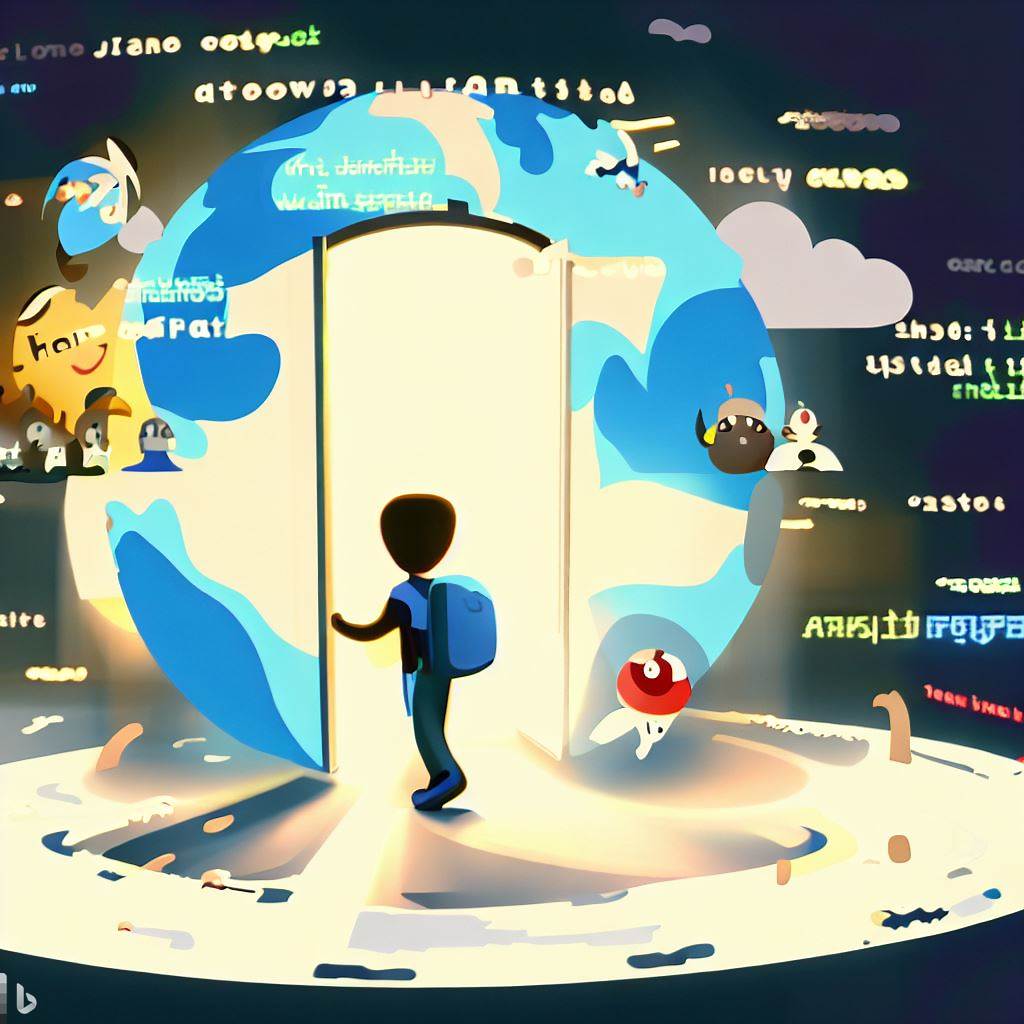
Getting Started
Welcome to the world of Java programming! May your coding journey be filled with excitement and success. Java, a versatile and powerful language, is perfect for building applications, websites, and more. Begin by grasping core concepts like variables, loops, and functions. Explore object-oriented programming to create efficient and modular code. Your dedication will surely lead to mastery. Best wishes on your Java adventure!

Guide
Dive into Java programming with this concise guide. Learn syntax essentials, data types, and control structures. Explore object-oriented principles for building efficient applications. Harness the Java standard library for pre-built functions. Master input/output and exception handling. Strengthen skills with practice and online resources. Enjoy your coding journey and may your Java endeavors be rewarding and full of growth!